Exercise notebook: Git committing
The notebook builds on our peer-reviewed pedagogical foundations.
We your feedback and suggestions on this notebook!
With this notebook, you can practice committing changes in Git.
Practice | Label | Time (min) |
---|---|---|
1 | Clone the repository | 5 |
2 | Create, stage, and commit changes | 10 |
3 | Undo committed changes | 8 |
4 | Create Atomic commits | 10 |
5 | Undo changes (advanced) | 5 |
6 | Wrap-up | 2 |
Overall | 40 |
We are here to help if errors or questions come up!
Part 1: Set up the repository
Task: Start GitHub Codespaces from the CoLRev repository.
Note: To create an empty git project, you would run git init
.
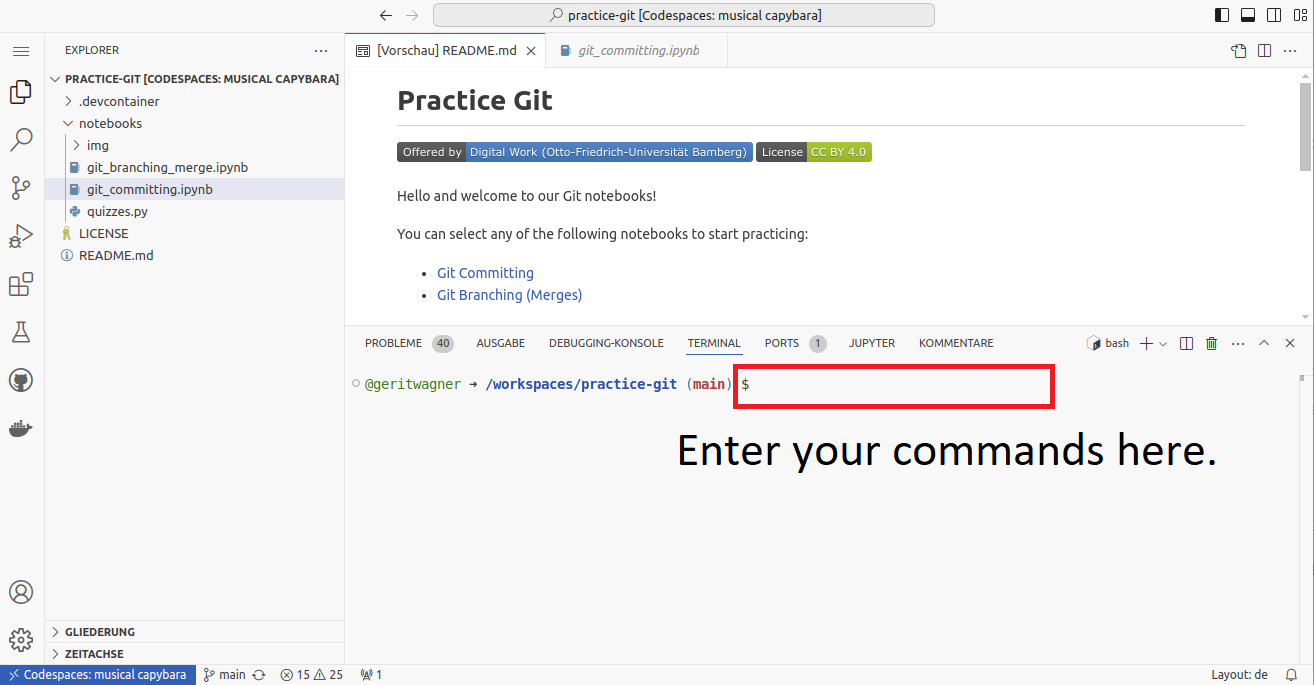
The status command provides an overview of the current state of the project and the files in the three sections. Therefore, you will need to run git status
regularly.
Note: The comments after the hashtag (#) are ignored.
# Check the status of the project
git status
Check
The git status
command should print something like the following:
On branch main
Your branch is up to date with 'origin/main'.
nothing to commit, working tree clean
The last line indicates that there are no changes in the staging area (nothing to commit). The working directory has the same content as the last version in the git repository (working tree clean). Part 2: Create, stage, and commit changes
Next, we modify files (state: untracked/modified), mark them to be in the next commit (state: staged), and create the first version (state: committed). This corresponds to the three sections of a Git project.
Task: Open the README.md
file in the colrev repository (shift
+ double click to open in a separate tab) and add your name to the project citation (# Citing CoLRev
section).
# Check the `git status` between each command
git status
Task: Open the CONTRIBUTING.md
file and change it.
The git status
should now show two files with changes in the working directory (state: modified)
We decide that the changes in the README.md
file should be staged for the next commit. The changes in the CONTRIBUTING.md
file are no longer needed.
Task: Use the commands suggested by git status
to accomplish this.
This means that changes in the README.md are staged (to be committed).
Check
The git status
should now display:
On branch main
Your branch is up to date with 'origin/main'.
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: README.md
To commit the changes, we run
git commit -m 'add contributor'
The -m 'add contributor'
adds a short summary message, which is expected for every commit.
Check
git status
should reflect your expected state of files in the three Git sections. Part 3: Undo committed changes
To undo the last commit, we can simply run:
git reset --soft HEAD~1
You should now have the README.md
file in the staging area again.
Note: the HEAD~1
refers to the last commit.
Task: Run git status
to see the changes.
We decide to discard our changes.
Task: Use the commands suggested by git status
to do that.
Check
The git status
should show the following:
On branch main
nothing to commit, working tree clean
To analyze the specific changes, open the Git GUI:
Part 4: Create atomic commits
It is good practice to create atomic commits, i.e., small changes that belong together. One should avoid large commits that modify many unrelated parts of the code base and pursue different objectives.
Analyze the following commits and discuss which ones are atomic and which ones combine changes that do not belong together (i.e., should be in separate commits). In addition, check the commit message (short summary at the beginning). Does the message clearly summarize the changes?
Solution
Atomic commit, ok.Solution
Relatively atomic. There are a few changes beyond `compute_language()`. May be improved.Solution
Many files changed. Changes not related to each other. Message refers to refactoring and testing, but the commit also adds functionality.Solution
Many files changed, but the changes belong together. ok.Solution
Atomic commit, ok. It is ok to combine functionality, tests, and docs that belong together in one commit!Optional: If you have the time, you may check the Conventional Commits specification.
To create atomic commits, you may need to add specific lines of code that should go into a commit, leaving other changes in the working directory.
The changes are provided in the rec_dict.patch file, which must be placed in the project’s working directory. To apply it, run:
# Suggests to rename the method but also introduces unrelated changes.
git apply rec_dict.patch
# Different files were modified by the patch
git status
Task: Use the Git GUI to check the changes that were introduced by the patch.
In the following, we would like to add only the changes in lines related to the load_records_dict
method and the skip_notification
parameter (using -p
for a partial git add
):
# Add specific lines of code from the colrev/dataset.py
# using y/n to add or skip (confirming with ENTER)
git add -p colrev/dataset.py
Task: Check whether the correct lines were added! Create a commit containing the relevant changes. Afterward, discard the remaining changes.
Part 5: Undo changes (advanced)
To undo committed changes, there several options:
- Revert the commit, i.e., create a new commit to undo changes:
git revert COMMIT_SHA --no-edit
- Undo the commit and leave the changes in the staging area:
git reset --soft COMMIT_SHA
(*) - Stage changes, and run
git commit --amend
to modify the last commit (*)
If you have the time, try the different undo operations in the session.
(*) Important: only amend commits that are not yet shared with the team. Otherwise, a revert is preferred.
Even if you run
git reset --hard ...
, you can still recover commits using git reflog
. Committed data will only be lost permanently if you run git reflog expire --expire=now --all
and git gc --prune=now --aggressive
. If the commits are already on GitHub, you would need git push --force
and the changes may also be synchronized in other local repositories. Avoid options like
--force
, --hard
, or --aggressive
. Use them only if you know what you are doing. Wrap-up
🎉🎈 You have completed the Git commit notebook - good work! 🎈🎉
In this notebook, we have learned to
- Clone a repository and check the
git status
- Create, stage, and commit changes using
git add
,git commit
andgit restore
- Create atomic commits
- Undo changes
- Navigate VisualStudio Code on GitHub Codespaces
Remember to delete your codespace here (see instructions).